
Limbo
Limbo has been sitting on my Xbox 360 for the past few months, and I finally took the time to sit down and experience it in its entirety. I quickly found myself deeply engrossed in its foreboding atmosphere, clever puzzles, and unconventional storytelling. It is one of those games that pushes the medium forward and offers a glimpse of how the interactive medium can evoke emotions in such a way as to rival the most moving pieces in other art forms.
The first thing I noticed about Limbo is its unique visuals. It portrays a dim, black and white world that uses focus and shadows to create a sense of depth and mystery. The animations of your character portray a scared, weak little boy who is unable to defend himself. The effect is compounded with large, aggressive enemies and gruesome death sequences. These all combine to create an ominous environment that never feels safe and leaves you nervous about every step.
Limbo’s sound is just as effective at creating an engaging atmosphere. The boy’s footsteps echo in a near-silent forest, leaving you unsure of what you might find next. The stillness is shattered with the loud crashes of attacking creatures or smashing boxes, coupled with strong, sudden vibration from the controller. This brings you further to the edge of your seat as you avoid dangers, and downright scares you in some cases.
In many ways, Limbo’s presentation of ideas and expressions through gameplay is exemplary. It leads your emotions not only through the visual and auditory feedback you receive, but also through the movement of your character and the actions you perform. Read on for my interpretation of what this is saying, and how it communicates without a single piece of dialog.
WARNING: Some spoilers below. Due to the importance of surprise in some cases, I’d advise you to play before you continue reading.

Fear
The word “limbo” has a great deal of religious connotations, but I don’t interpret this game as a story about a boy existing somewhere between Heaven and Hell. Rather, it is a metaphor for the idea of being stuck in a situation or state of mind, unable to make progress outward. A fitting substitution would be “The Waiting Place”, as referenced in Dr. Suess’ brilliant Oh! The Places You’ll Go (forgive the reference – I’m a dad; this is one of my daughter’s favorite books). Here’s an excerpt:
“The Waiting Place…for people just waiting.
“Waiting for a train to go or a bus to come, or a plane to go or the mail to come, or the rain to go or the phone to ring, or the snow to snow or waiting around for a Yes or No or waiting for their hair to grow. Everyone is just waiting.
“Waiting for the fish to bite or waiting for wind to fly a kite or waiting around for Friday night or waiting, perhaps, for their Uncle Jake or a pot to boil, or a Better Break or a string of pearls, or a pair of pants or a wig with curls, or Another Chance. Everyone is just waiting.”
Limbo is a journey out of The Waiting Place.
The first thing that you do in Limbo is make the decision to act. When you start the game, the boy is lying on the ground, motionless. Only when you choose to act does the adventure begin. If you choose not to, the boy stays trapped in limbo. There is no escaping The Waiting Place without the desire to act. This first button press sets the stage for the rest of the game – your actions are specifically designed to elicit emotions or ideas from the game.
The first area is a dense forest, wherein we find our first adversary: the spider, which represents our fears. Spiders are typically small creatures, but this spider is massive and seemingly impossible to overcome. Fears often seem larger and more daunting than they are, and this enemy certainly reflects that. Overcoming these fears will not be easy. You begin by timidly facing the spider by moving towards it to provoke it, then running away as it attacks. You are then stuck in its web and prepared as its meal, reflecting the danger of letting fears limit us – to the point that we cannot move and are consumed by them. Finally, the game forces you, by means of an approaching boulder, to stop running from the spider and face it head on in a moment of absolute terror: you then find that this spider is not so indestructable. By the end, you face a defeated, pitiful enemy and even use it as a means to progress toward the exit of The Waiting Place.
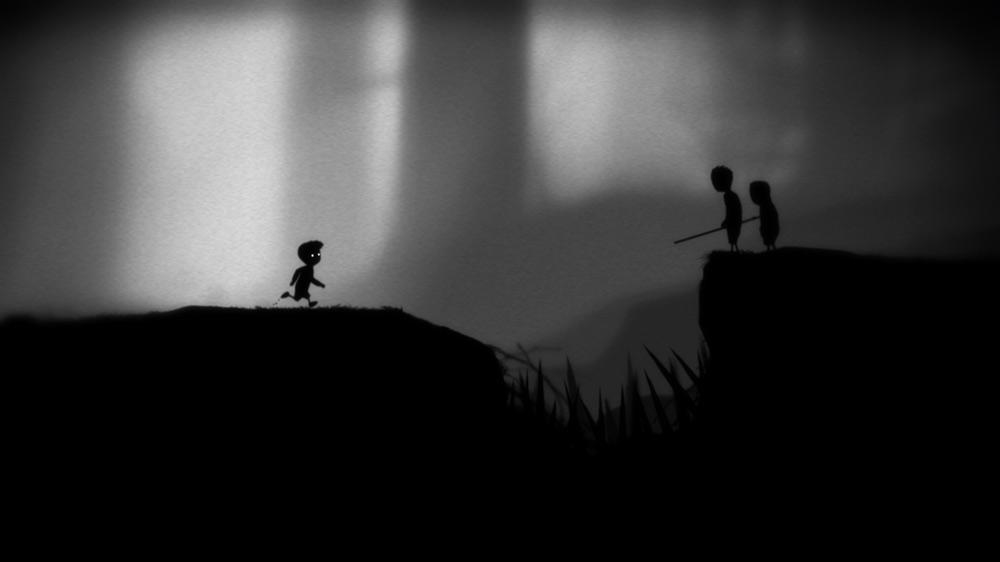
Facing our Peers
You are then thrust into the midst of a group of hostile kids, a representation for the peers that limit us to where we are. This could be active on their part, such as when one of them manipulates the boy’s fear with a mechanical spider look-alike. It could also be our own concern for what others think. This is portrayed a few times when the others simply stand in front of an obstacle, staring at the boy, seemingly saying “you can’t do this”. There are other boys hung amongst the trees, discouraging you with the failures of others. You continue to push forward, ignoring their attempts to stop you. Finally, these adversaries are destroyed as a result of their attacks against you.
You then enter a city and stumble upon a dilapidated hotel sign. Hotels remind me of rest and finding comfort, but the sign for the hotel points to a pit that would result in the boy’s death. This seems to convey that, if we are to get out of The Waiting Place, we can’t get comfortable. We have to push forward. After passing the hotel, you fall into a factory, where you are pushed along conveyor belts and gears. This area represents the habits and routines that keep you in limbo. Staying on the conveyor belt and following the gears leads to death; instead, you must choose a different path. You must choose to break those habits and accept change.
In the final areas, the shifting of gravity and magnetic attractions is key to the puzzles you must overcome. You fall down, then fall up, and must change gravity in mid-air to reach your intended destination. This represents the obliterated confidence you have when stuck somewhere. The gravity shifts from moment to moment as you second-guess yourself and remain unsure of what is up and down.
Finally, after overcoming your fears, what others think, your self-destructive habits, and lack of confidence, you shatter through the glass of The Waiting Place and find yourself…back in the forest, lying on the ground.
Once again, you choose to get up and start moving forward. Are you still stuck? Are you right back where you started?
No. Something is different this time.
This time, you find what you were looking for. You are in the same place you started, sure, but you are not the same person. You know the way out, and you know who the people are that matter most – those who will help you get there.
Want some other thoughts? Check out GamesRadar’s compilation of interpretations of Limbo from their writers. There’s some really insightful thoughts in some of those.